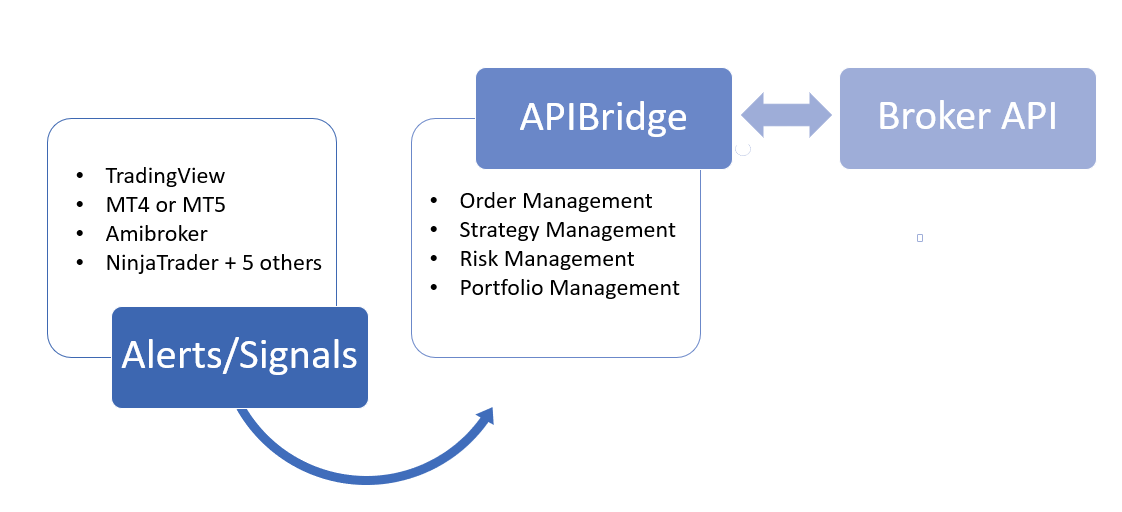
Signal parameters are of 4 types: Long Entry (LE), Long Exit (LX), Short Entry (SE), Short Exit (SX).
Easiest – Zero Programming Effort
- Google Chrome Extension to fetch & process alerts with free TradingView Subscription
- Moreover Webhooks which work with TradingView and other platforms
- Finally Excel: Only simple formula is sufficient to setup strategies.
Intermediate – Coding in Scripts
- Amibroker: AFL codes for adding to strategy, dll plugin files
- MT4: Mq4, ex4 and mqh files
- NinjaTrader: ninjascript files
- Excel: VBA Macros
Advanced – Programming
Python/C#/Java: Above all Programming the entire strategy and sending data to APIBridge
Signal Format & Coding Guide
Bridge allows you to customize all values at each signal, for instance quantity, or order type. Although you can program your own strategy to place orders with all custom values at each signal. Secondly for controlling order params via code, you should leave blank values in the Bridge under Symbol Settings. Most importantly the indication of mandatory values for each Symbol is with * under Symbol Settings.
The Signal format is:
ID,Type,Symbol,OrderType,TrigPrice,Price,Qty,InstrumentName,StrategyTag
- ID (mandatory): should be unique id for each signal. In case if two signals are provided with same id, the application may misbehave.
- Type (mandatory): can be either LE, LX, SE or SX.
- Symbol (mandatory): symbol name which will be matched to Input Symbol underSymbol Settings. If you want to send strike/expiry also through Amibroker, you can combine it in syntax “Symbol | Expiry | Strike | OptionType”
- OrderType (optional): It can be Limit, Market, SLL or SLM. It can be combined for ProductType using “|”.
- TrigPrice (optional): should be 0 or Null for market/limit orders. Although If you want to send extra parameters for BO, you can combine it in syntax “TrigPrice | StopLoss | SquareOff | TrailingTicks”
- Price (optional): should be 0 or Null for market orders
- Qty (optional): Order Quantity
- InstrumentName (optional): It should be any of the following EQ|FUTIDX|FUTSTK|OPTIDX|OPTSTK|FUTCUR|FUTCOM
- StrategyTag (optional): It can be any string to identify which particular strategy is sending Signals. Advanced: you can also send signal to particular port in syntax “StategtTag | Port”. Example: “STG1|30002”
Do you want coding help to deploy your own strategy for live trading? Check our coding assistance.
Coding Examples
You can see below actual code samples in Amibroker, Connector and TradingView. In addition all examples given below are mostly send using blank columns in Bridge to place order.

1: Send Market Order in Equities to create Short position:
AFL Code: AlgoJi_Signal("1", "SE","SBIN","Market|MIS","","","100","EQ","STG1"); Text File string: 1,SE,SBIN,Market|MIS,,,100,EQ,STG1 TradingView Alert: TYPE:SE:SYMBOL:SBIN:OT:Market|MIS:QTY:100:INS:EQ:STAG:STG1
2: Send Stop Limit Order in Equities to create Short position:
AFL Code: AlgoJi_Signal("2", "SE","SBIN","SLL|MIS","302.50","302","100","EQ","STG1"); Text File string: 2,SE,SBIN,SLL|MIS,302.5,302,100,EQ,STG1 TradingView Alert: TYPE:SE:SYMBOL:SBIN:OT:SLL|MIS:TRIG:302.5:PRICE:302:QTY:100:INS:EQ:STAG:STG1
3: Send Stop Market Order in Equities to create Long position:
AFL Code: AlgoJi_Signal("8", "LE","SBIN","SLM|MIS","302","0","100","EQ","STG1"); Text File String: 8,LE,SBIN,SLM|MIS,302,0,100,EQ,STG1 TradingView Alert: TYPE:LE:SYMBOL:SBIN:OT:SLM|MIS:TRIG:302.5:PRICE:0:QTY:100:INS:EQ:STAG:STG1
4: Send Cover Order in Equities for Long trade:
AFL Code: Algoji_Signal("6", "LE","SBIN","Limit|CO","302|1.5","302.10","100","EQ","STG1"); Text File String: 6,LE,SBIN,Limit|CO,302|1.5,302.1,100,EQ,STG1 TradingView Alert: TYPE:LE:SYMBOL:SBIN:OT:Limit|CO:TRIG:302.5|1.5:PRICE:302.10:QTY:100:INS:EQ:STAG:STG1
5: Send Bracket Order in Equities for Long trade:
AFL Code: Algoji_Signal("7", "LE","SBIN","SLL|BO","302|1.5|3","302.10","100","EQ","STG1"); Text File String: 7,LE,SBIN,SLL|BO,302|1.5|3,302.10,100,EQ,STG1 TradingView Alert: TYPE:LE:SYMBOL:SBIN:OT:SLL|BO:TRIG:302|1.5|3:PRICE:302.10:QTY:100:INS:EQ:STAG:STG1
6: Send Limit Order in BankNifty Futures to create Short position:
AFL Code: Algoji_Signal("6", "SE","BANKNIFTY","Limit|NRML","","28400","20","FUTIDX","STG1"); Text File String: 6,SE,BANKNIFTY,SLM|MIS,,28400,20,FUTIDX,STG1 TradingView Alert: TYPE:SE:SYMBOL:BANKNIFTY:OT:SLM|MIS:PRICE:28400:QTY:20:INS:FUTIDX:STAG:STG1
7: Send Limit Order in BankNifty Options to exit Long position:
AFL Code: Algoji_Signal("6", "LX","BANKNIFTY|28Nov2019|28400|CE","Limit|NRML","","102.50","20","OPTIDX","STG1"); Text File String: 6,LX,BANKNIFTY|28Nov2019|28400|CE,Limit|NRML,,102.5,20,OPTIDX,STG1 TradingView Alert: TYPE:LX:SYMBOL:BANKNIFTY|28Nov2019|28400|CE:OT:Limit|NRML:PRICE:102.5:QTY:20:INS:OPTIDX:STAG:STG1
8: Send Market order in Currency Futures to exit Long position:
AFL Code: Algoji_Signal("6", "LX","EURINR|27Nov2019","Market|NRML","","0","1000","FUTCUR","STG1"); Text File String: 6,LX,EURINR|26Nov2019,Market|NRML,,0,1000,FUTCUR,STG1 TradingView Alert: TYPE:LX:SYMBOL:EURINR|26Nov2019:OT:Market|NRML:PRICE:0:QTY:1000:INS:FUTCUR:STAG:STG1
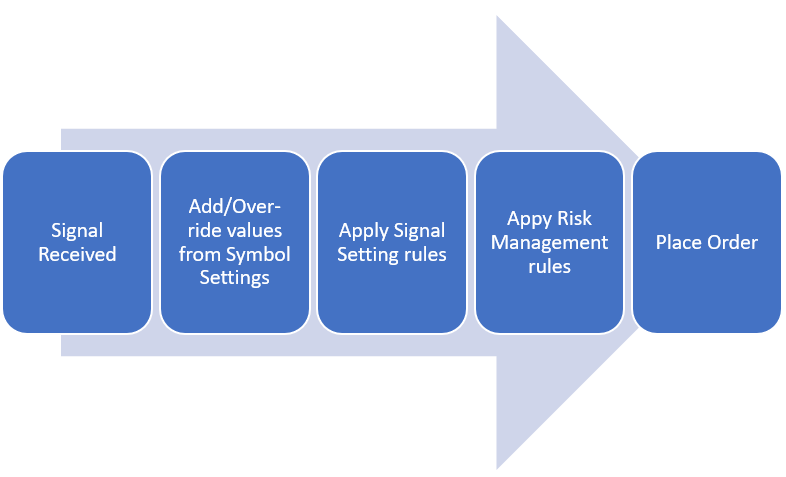
As per above process diagram, the sequence of Signal processing is as follows:
- Firstly signal is received in Bridge
- Secondly values are taken from Symbol Settings window by matching symbol given in Signal with “Input Symbol”. Also values in Symbol Settings over-ride that given in Signal. For example, if qty in Symbol Setting is 100 and Signal received is 200, Bridge will place order with 100 qty only. If Qty is blank/null in Symbol Settings, the it will place the Qty from Signal (200 in this case).
- Thirdly Signal Rules are applied from Application Settings -> Signal Rules
- After that Risk Management rules are applied.
- Finally order is sent to your Broker API
Do you want coding help to deploy your own strategy for live trading? Check our coding assistance.
Hi Team,
I m facing issue with api bridge – chart bridge setup. I m using Trading view 5 mins candle, and most of days, I get Object reference not set.. error repeatedly during day. Now, I m using same alert format, and sometimes it works and sometimes it doesnt. When this error comes, no more trade happens from Trading View.
Can you please help me how can I check this.
Thanks
did you try the steps here? http://mycoder.pro/apibridge/object-reference-not-set-to-any-instance-of-any-object/
Hi, Can i place ATM Call or Put without specifying strike in bridge.Or any option to send strike , CE or PE info from trading view itself without modifying bridge settings.
This is needed because trading view in chrome & chartbridge is running in vps , i may set alert from tradingview mobile app without opening vps.
You can bulk import option strikes in apibridge, see here http://mycoder.pro/apibridge/how-to-import-export-symbol-settings/
Hi Team,
Yes, I did… see I m using api bridge to fetch signals from Trading view… they work sometimes, but then suddenly I get this error in chart bridge. So it cannot be that signal format is wrong..
If I have 5 layouts in Trading view, and I get LX from all 5, then mostly I have seen this error.
Even if it work some times, your symbol settings could be wrong. Please try again with only 2-3 symbol rows. Once you are confident on symbol rows, you can export them – to add as many as you wish – then import it again.
is maxprofit grouped at strategy level? for example if I have created straddle using combination of two strikes and tagged it as STG2 with max profit amount of 2000 so will it take combined profit of both the strike or individual strike?
no, maxprofit works only at trade-basis for the particular symbol. It is independent of strategy tag.
There is issue with application…. how really does 5 symbol or 3 symbol matter?
I dont have any max profit at strategy level, its at symbol level…
5 symbol or 3 symbol matter because its easier to diagnose which setting is wrong. The application is tested and recommended by many third-party companies. So we can assure you there is no problem with application.
I worked with only HDFC… it was still same issue… noticed over three sessions…
Hi Can we trade with apply stop function of amibroker. Which would normally not generate sell or cover signal. But as mentioned in amibroker forum web site if we apply equity(1) function, it will also create sell, cover signal also. So can we trade with apply stop function ?
applystop() does not generate sell or cover signal for real-time trading. You need to trigger Buy/Sell/Short/Cover in real-time. These sample AFLs can help http://mycoder.pro/apibridge/how-can-i-modify-afl-strategy-without-any-coding/
How to modify the existing SL order created using Cover Order.
My requirement is
I want to create a cover order (with SL).once the cover order executed, that means automatically stop loss order will be created.
Now i want to change the stop loss order as my stop loss price will change based on the stock movement.
Now question is How to send a modify order?.
You cannot exit/modify BO/CO via apibridge http://mycoder.pro/apibridge/exiting-bracket-orders-and-cover-orders/
Hi Team,
Can you please help in setting up Chartbridge for Crude Oil, MCX. I m not able to do that. What will be symbol settings.
Thanks
I am getting error ” CRX_REQUIRED_PROOF is missing. Kindly help
I want to place a stop loss order as ref(low,-2) whatever the price is while placing the order. I tried but it asks for a number. please guide.
Something like
AFL Code: AlgoJi_Signal(“8”, “LE”,”SBIN”,”SLM|MIS”,”ref(low,-2)”,”0″,”100″,”EQ”,”STG1″);
or
SLBuy=ref(low,-2)
AFL Code: AlgoJi_Signal(“8”, “LE”,”SBIN”,”SLM|MIS”,”SLBuy”,”0″,”100″,”EQ”,”STG1″);
The above do not work with or without quotes.
Example 5: Send Bracket Order in Equities for Long trade:
TradingView Alert: TYPE:LE:SYMBOL:SBIN:OT:SLL|BO:TRIG:302|1.5|3:PRICE:302.10:QTY:100:INS:EQ:STAG:STG
This TradingView Alert example of a Stop Limit (SLL) Bracket Order (BO) does not generate the correct Bracket Order on Interactive Brokers. It generates a Limit Order Bracket Order on Interactive Brokers. The coding for this needs to be checked and tested by the developers.
I have shown this problem to the Support team who are going to check with the developer if the mapping of SLL|BO is incorrect.
When using Connector for sending in signals, you also need to put another parameter (port number) at the end of the text file format. So for example if this the intended signal:
Text File String: 7,LE,SBIN,SLL|BO,302|1.5|3,302.10,100,EQ,STG1
then it should read
Text File String: 7,LE,SBIN,SLL|BO,302|1.5|3,302.10,100,EQ,STG1,3007
Port at the end of connector app is required only if you are not using the default port. Only our advance users change the port.
Please clarify,
i want to send an Equities Buy Limit BO for Amibroker, with only SL & Target, but without any trailing stop loss.
my code is : Algoji_Signal(“7”, “LE”,”SBIN”,”L|BO”,”0|1.5|3″,”302.10″,”100″,”EQ”,”STG1″);
Everything works fine, except somehow, am getting training SL, cause the initial SL keeps going up as the LTP keeps going up.
i want to avoid this situation. Kindly advise. TY.
MT4 setup done as per video but still order not place in api bridge and also no error found. then how can i place order. and also for alice blue i not found api credientails in mycoder.pro any where. can you help me for api credientails and MT4 setup